DJango 学习(5)—— Django 静态文件配置 以及 简单操作
静态文件配置
"""
html 文件默认放在 templates 文件夹下
网站所使用的静态文件默认放在 static 文件
静态文件
前端已经写好的 能够直接调用使用的文件
网站写好的 js 文件
网站写好的 css 文件
网站用到的图片文件
第三方前端框架
...
拿来就可以直接使用
"""
一般情况下我们在 static 文件夹内还会进一步的划分处理
-- static
-- js
-- css
-- img
-- other
"""
在浏览器中输入 url 能够看到对应的资源
是因为后端提前开设了该资源的接口
如果访问不到资源,说明后端没有开设该资源的接口
"""
STATIC_URL = '/static/'
"""
/static/others/JQuery3.6.0.js
这是的 /static/ 不是指文件夹,而是指 “令牌”
"""
STATICFILES_DIRS = [
os.path.join(BASE_DIR, "static")
]
"""查找顺序,从上往下一次查找,并且找到一个就停止查找"""
"""
当你在写 django 项目的时候 可能会出现后端代码修改了但是前端页面没有变化的情况
1. 你在同一个端口开了好几个 django 项目
一直在运行的其实是第一个 django 项目
2. 浏览器缓存问题(谷歌为例)
settings
network
disable cache 勾选上
"""
{% load static %}
<script src="{% static 'others/JQuery3.6.0.js' %}"></script>
<link rel="stylesheet" href="{% static 'others/bootstrap-3.4.1-dist/css/bootstrap.min.css' %}">
<script src="{% static 'others/bootstrap-3.4.1-dist/js/bootstrap.min.js' %}"></script>
"""
form 表单 action
1. 不写,默认当前页面所在的 url 提交数据
2. 全写
3. 只写后缀 /login/
"""
MIDDLEWARE = [
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
]
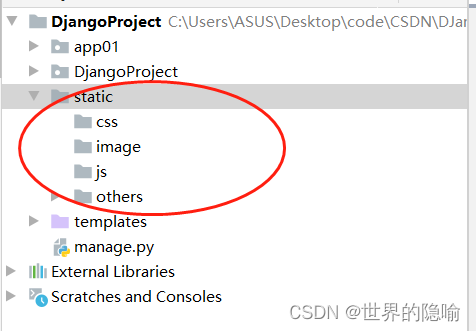
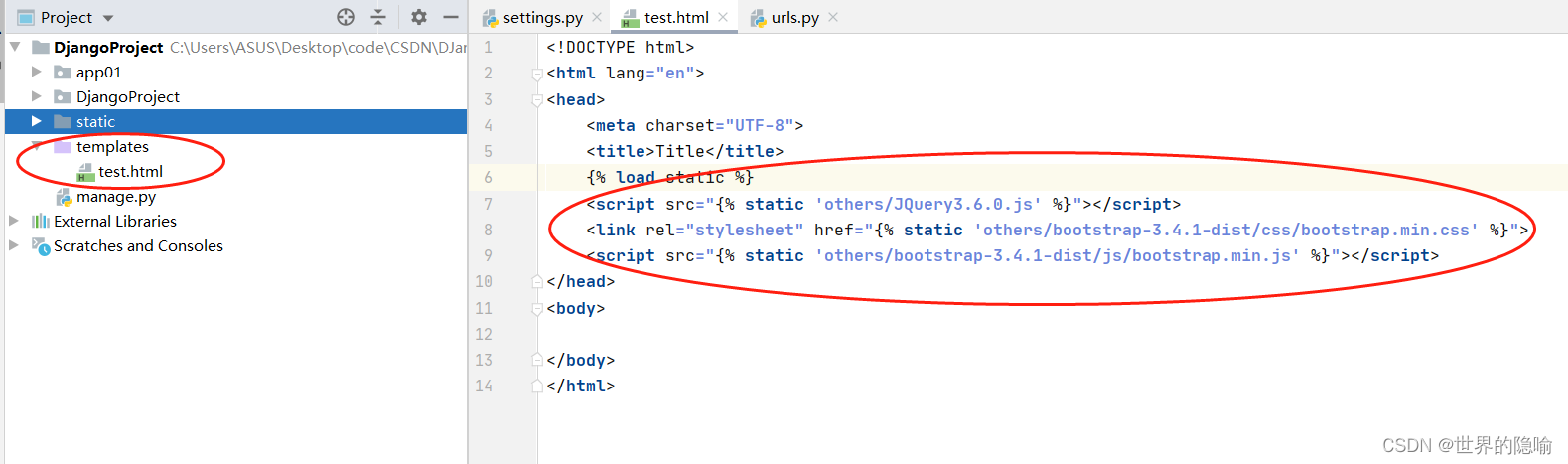
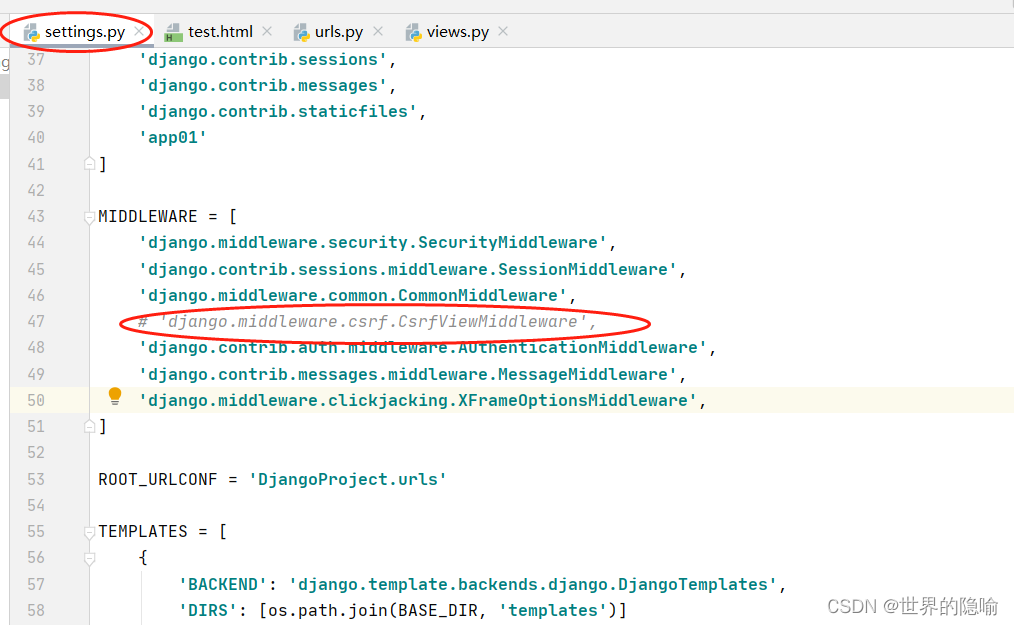
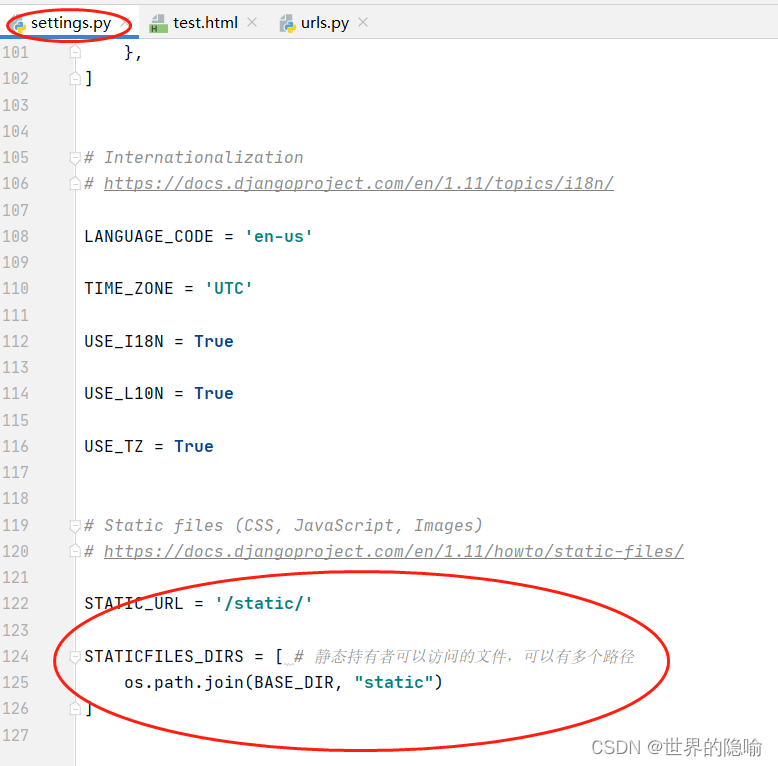
django 小白必会三板斧
"""
HttpResponse: 返回字符串
render: 返回 html 文件
# 第一种传值方式:更加精确,节省资源
# return render(request, "myFirst.html", {"data": user_dict})
# 第二种传值方式:当你要传的数据特别多的时候
"""locals 会将所在的名称空间中所有的名字全部传递给 url 页面"""
return render(request, "myFirst.html", locals())
redirect:重定向
return redirect("https://www.baidu.com")
return redirect("/home/")
"""
request 对象方法
request.method
request.POST
request.POST.get()
request.POST.getlist()
request.GET
def login(request):
"""
get 请求和 post 请求应该有不同的处理机制
:param request: 请求相关的数据对象 里面有很多简易的方法
:return:
"""
if request.method == "POST":
print(request.POST)
username = request.POST.get("username")
print(username, type(username))
hobby = request.POST.get("hobby")
print(hobby, type(hobby))
username = request.POST.getlist("username")
print(username, type(username))
return HttpResponse("收到了")
return render(request, "login.html")
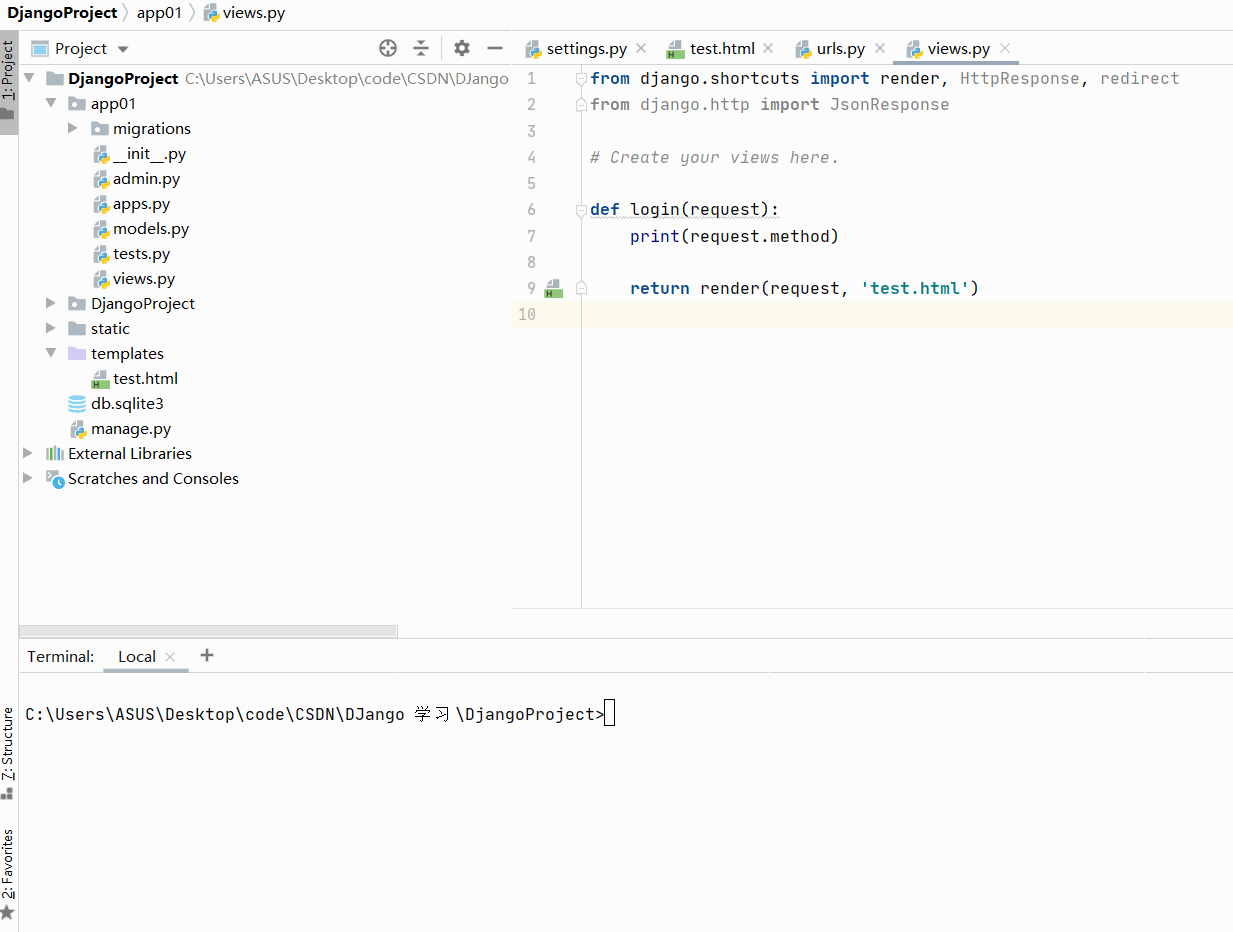
运行
login.html
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
{% load static %}
<script src="{% static 'others/JQuery3.6.0.js' %}"></script>
<link rel="stylesheet" href="{% static 'others/bootstrap-3.4.1-dist/css/bootstrap.min.css' %}">
<script src="{% static 'others/bootstrap-3.4.1-dist/js/bootstrap.min.js' %}"></script>
</head>
<body>
<h1 class="text-center">登录</h1>
<div class="container">
<div class="row">
<div class="col-md-8 col-md-offset-2">
<form action="" method="post">
<p>username: <input type="text" name="username" class="form-control"></p>
<p>password: <input type="password" name="password" class="form-control"></p>
<p>
<input type="checkbox" name="hobby" value="111">111
<input type="checkbox" name="hobby" value="222">222
<input type="checkbox" name="hobby" value="333">333
</p>
<input type="submit" class="btn-success btn-block">
</form>
</div>
</div>
</div>
</body>
</html>
views.py
from django.shortcuts import render, HttpResponse, redirect
from django.http import JsonResponse
def login(request):
if request.method == "GET":
print("你来了")
return render(request, "login.html")
elif request.method == "POST":
print("收到!!!")
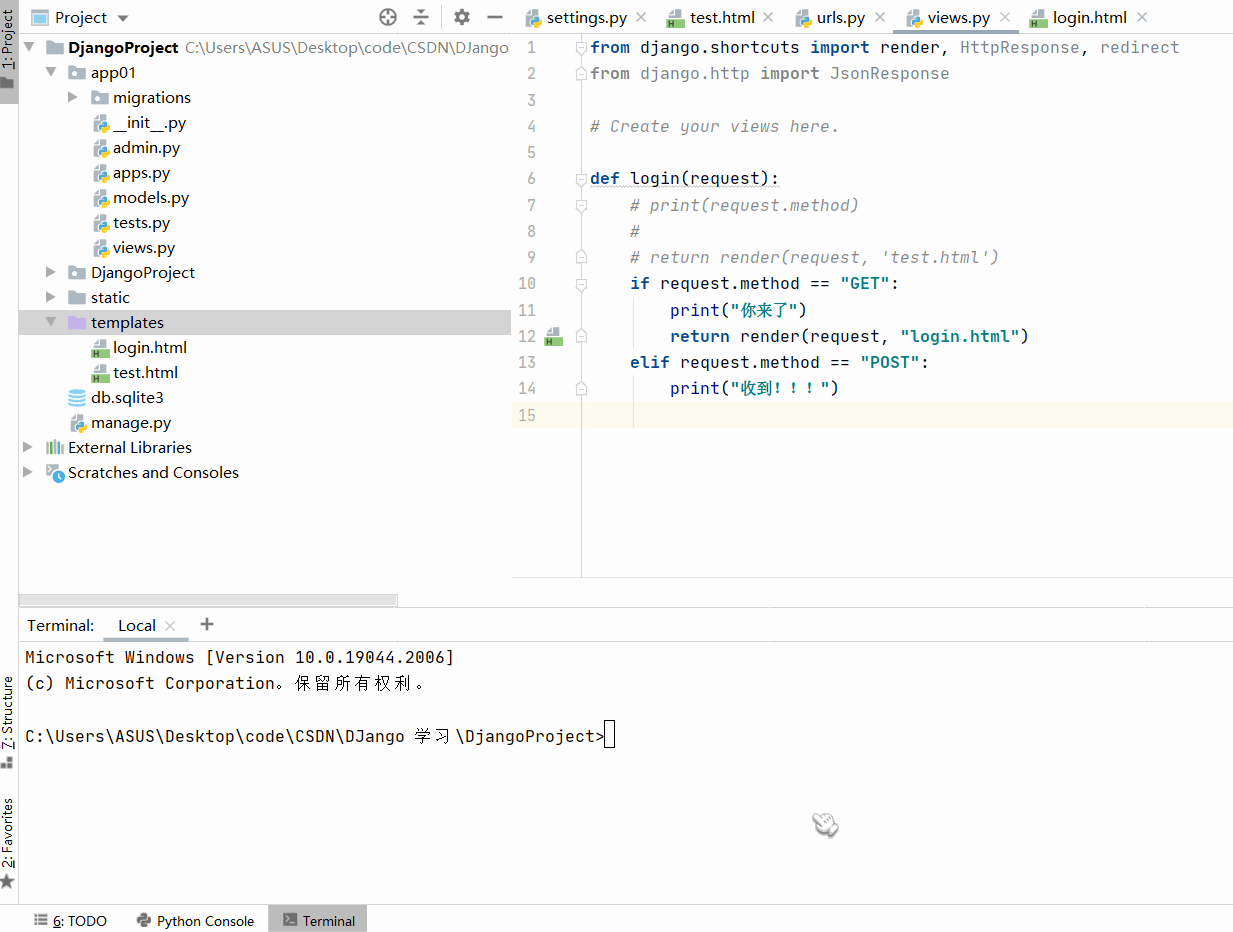
views.py
if request.method == "POST":
print(request.POST)
username = request.POST.get("username")
print(username, type(username))
hobby = request.POST.get("hobby")
print(hobby, type(hobby))
username = request.POST.getlist("username")
print(username, type(username))
return HttpResponse("收到了")
return render(request, "login.html")
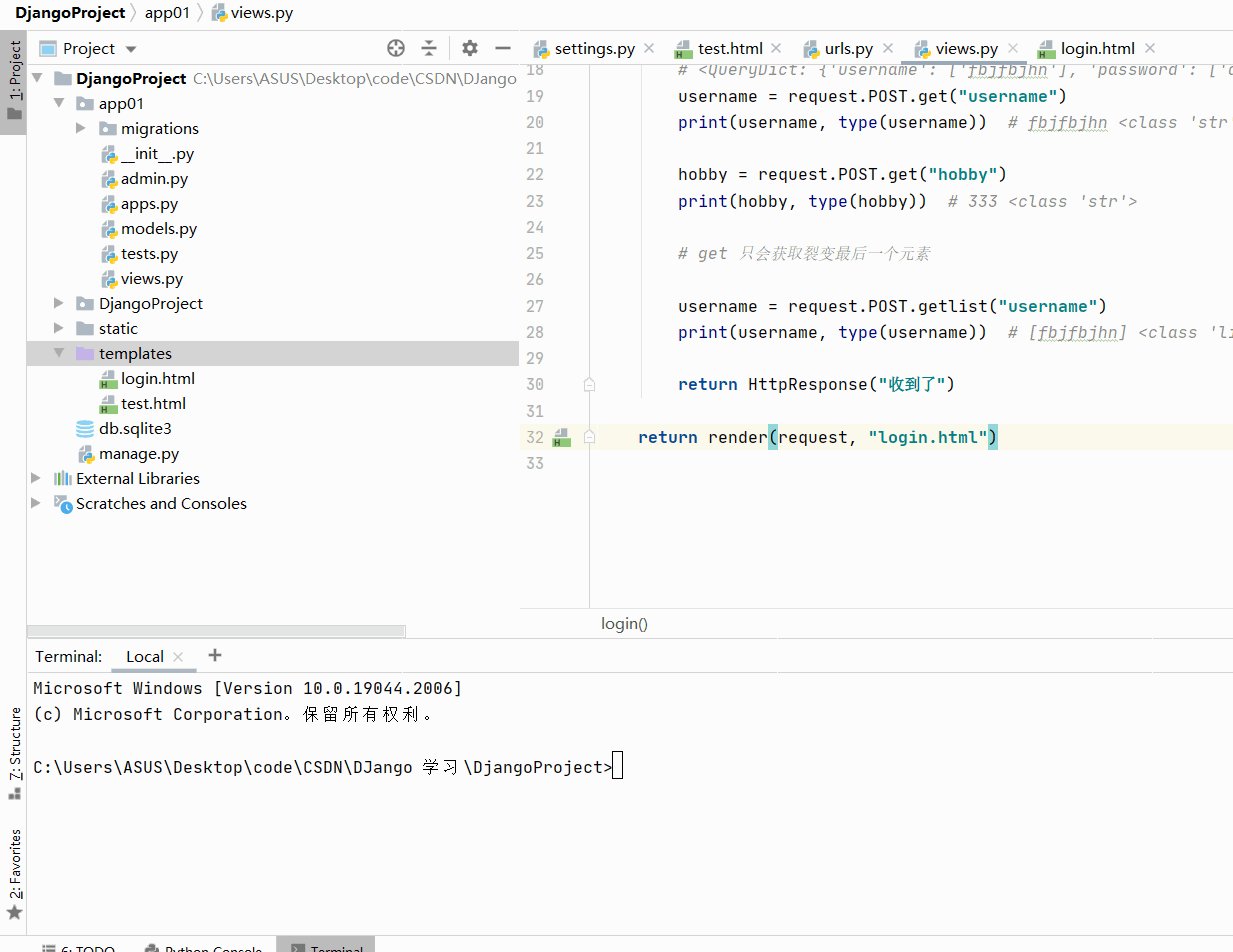