pymysql 基本语法及游标移动
import pymysql
conn = pymysql.connect(
host="127.0.0.1",
port=3306,
user = "root",
password="",
database="test",
charset="utf8",
)
"""
+------+--------------+
| id | name |
+------+--------------+
| 200 | 技术 |
| 201 | 人力资源 |
| 202 | 销售 |
| 203 | 运营 |
| 205 | sale |
+------+--------------+
"""
cursor = conn.cursor(cursor=pymysql.cursors.DictCursor)
sql = "select * from dep"
"""
+------+--------------+
| id | name |
+------+--------------+
| 200 | 技术 |
| 201 | 人力资源 |
| 202 | 销售 |
| 203 | 运营 |
| 205 | sale |
+------+--------------+
"""
res = cursor.execute(sql)
print(res)
print(cursor.fetchone())
print(cursor.fetchone())
cursor.scroll(1, "relative")
print(cursor.fetchone())
cursor.scroll(1, "absolute")
print(cursor.fetchall())
sql 注入以及解决方法
sql 注入
create table user (
id int primary key auto_increment,
name char(16),
password varchar(32)
);
insert into user(name, password) values
("aoteman", "asd"),
("alterman", "asdzxc");
import pymysql
conn = pymysql.connect(
host="127.0.0.1",
port=3306,
database="test",
user="root",
passwd="",
charset="utf8"
)
"""
+----+----------+----------+
| id | name | password |
+----+----------+----------+
| 1 | aoteman | asd |
| 2 | alterman | asdzxc |
+----+----------+----------+
"""
cursor = conn.cursor(cursor=pymysql.cursors.DictCursor)
while True:
username = input("username:>>")
password = input("password:>>")
sql = "select * from user where name='%s' and password='%s' " % (username, password)
print(sql)
cursor.execute(sql)
print(cursor.fetchall())
正常登录情况
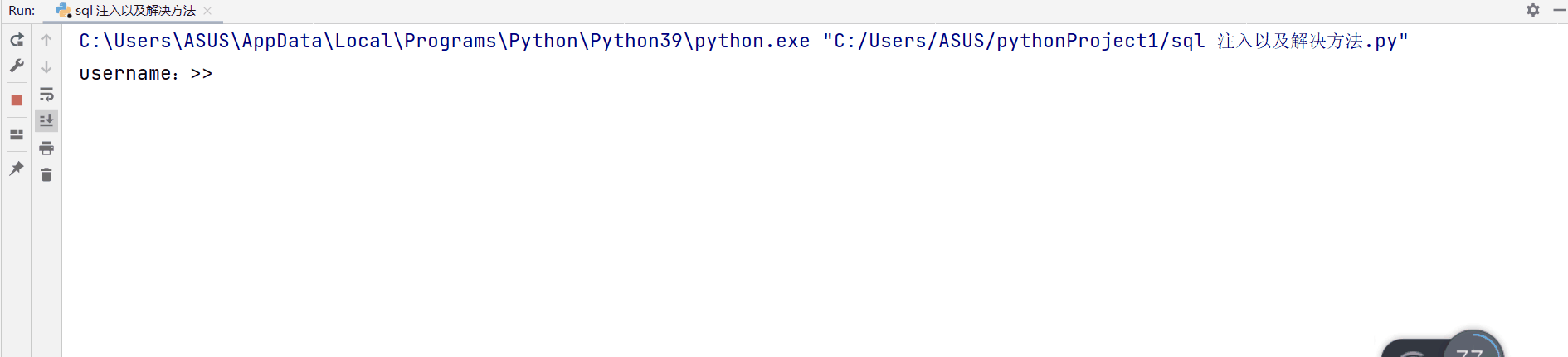
非正常登录情况
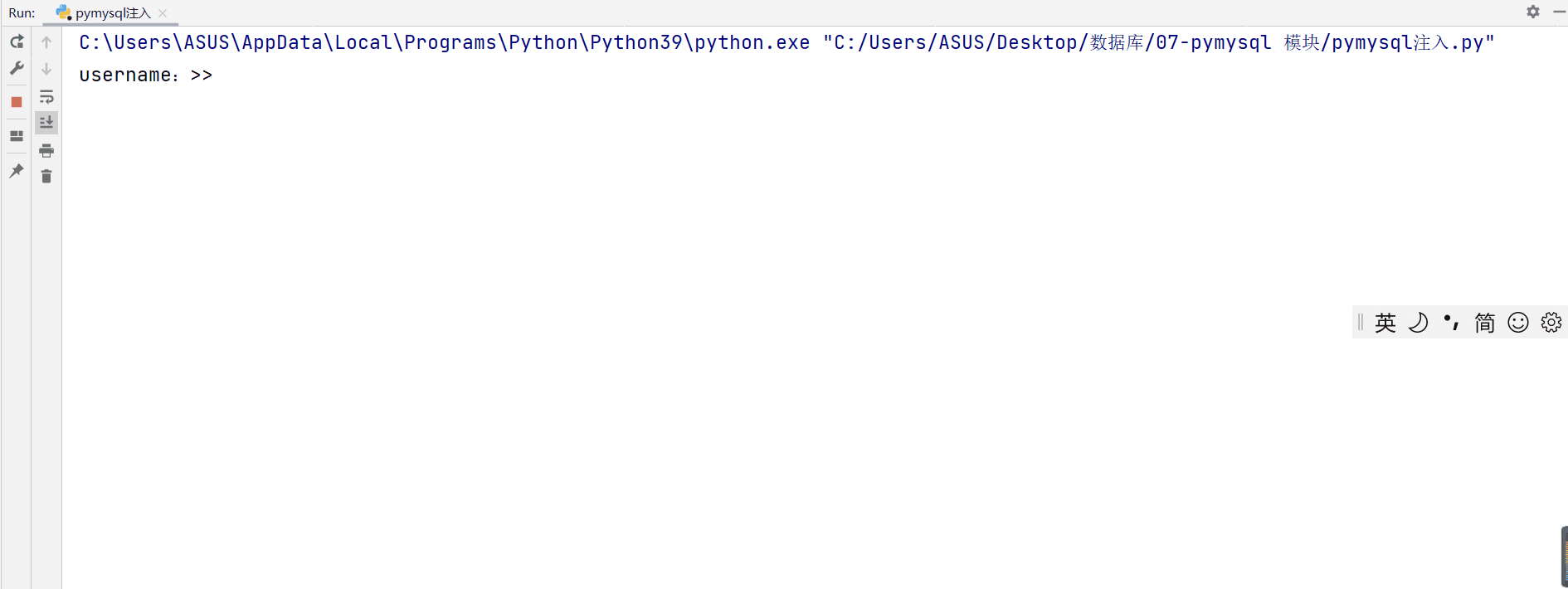
'''
在上述演示的非正常登陆情况下,我们发现
一种是只知道 用户名就可以实现登录,并且该登录用户的返回密码
一种是不知道账号和密码的情况下,完成登录,并且获取所有用户的用户名和密码
'''
我们在分析 mysql 在上述两种情况中执行的代码分别是
select * from user where name='aoteman'
select * from user where name='' or 1=1
分析执行的 sql 语句我们会发现这些非正常登录的情况是因为 用户巧妙运用了 mysql 的逻辑运算(or)和 注释符号 “
数据库注入情况是比较常见的,比如说我们在注册一些账户的时候,会要求我们的用户名和密码不能包含一些特殊符号就是为了防止注入的情况出现。
sql 注入的解决方法
sql = "select * from user where name=%s and password=%s "
cursor.execute(sql, (username, password))
在演示中我们可以看到按照这种方法可以比较简单的避免 sql 注入的情况出现
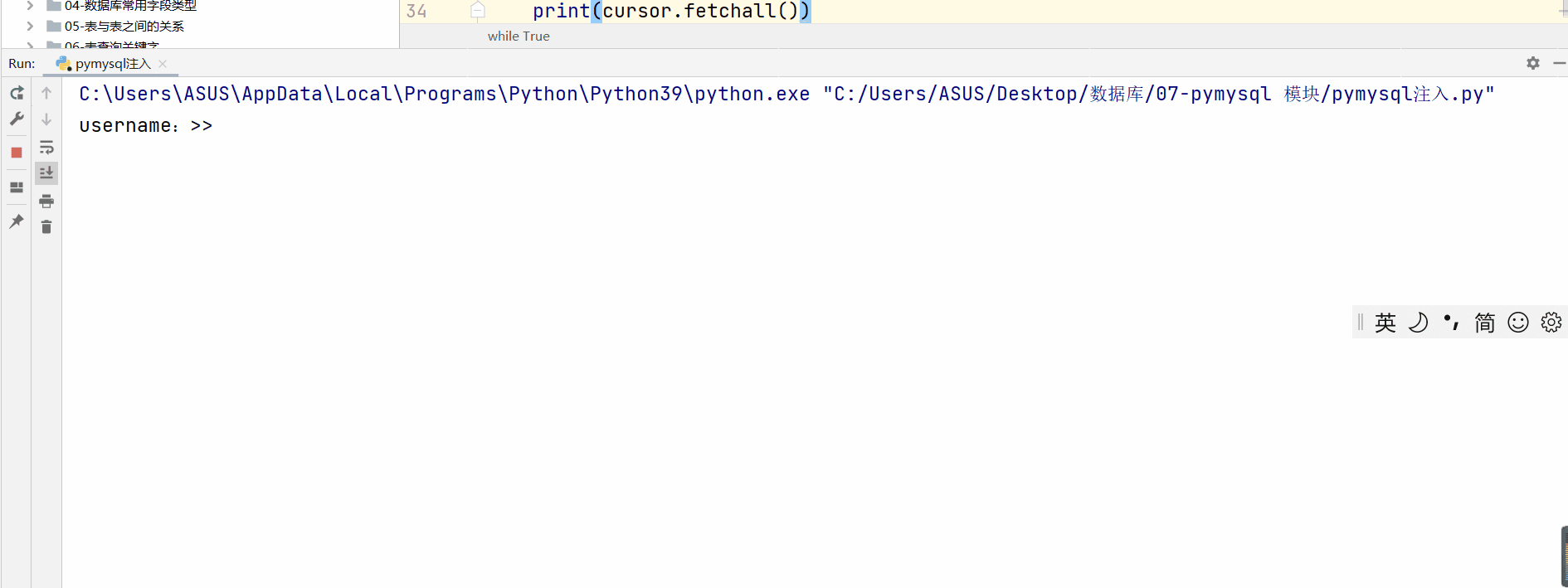
pymysql补充内容
'''
增删改查中
删、改、增它们的操作涉及到数据的更改,没有办法直接执行,需要二次确认
'''
rows = cursor.executemany(sql, [("sekiro", "123"), ("ash", "123456")])
import pymysql
conn = pymysql.connect(
host = "127.0.0.1",
port = 3306,
user = "root",
password = "",
charset = "utf8",
database = "test518",
autocommit = True
)
cursor = conn.cursor(cursor = pymysql.cursors.DictCursor)
sql = "insert into user(name, password) values(%s, %s)"
rows = cursor.executemany(sql, [("sekiro", "123"), ("ash", "123456")])
print(rows)
sql = "update user set name='ash' where id = 1"
rows = cursor.execute(sql)
print(rows)
sql = "delete from user where id = 1"
rows = cursor.execute(sql)
print(rows)